In this article on Understanding PHP Syntax, I will explain how to write simple programs in PHP. Also, you will learn how to create variables in PHP, data types, operators, and expressions.
A Simple Program for Understanding PHP Syntax
The following code shows a simple program to display text in PHP.
<?php
echo 'Welcome to programmingempire.com!';
?>
Output

The above code shows the specified text on a web page. As can be seen, the PHP code is enclosed in
<?php and ?>. Each PHP statement terminates with a semicolon (;).
We can insert PHP code anywhere along with HTML. The following code shows an example.
<?php
echo 'Welcome to programmingempire.com!';
?>
<br/>
<h2>Visit <a href="https://www.programmingempire.com/" target="_blank">Programmingempire</a> for more detail!</h2>
Output
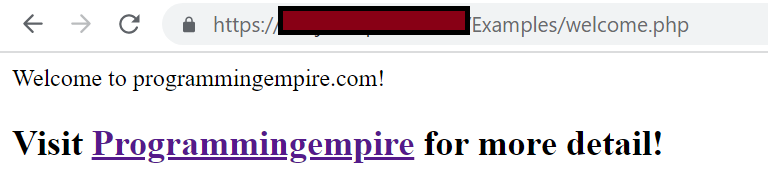
Like other programming languages, PHP code comprises keywords, variables, constants, operators, and strings. These are referred to as tokens of the PHP programming language. The following section briefly describes the tokens in PHP. Basically, a token is an independent element in a program.
Keywords
These are the reserved words that have some specific meaning. Therefore, while creating variables, and constants we can not use keywords. For instance, while, for, if are some examples of keywords.
Variables
Basically, variables hold values during the execution of a program. Furthermore, a variable can change its value during the execution. Each variable has a scope and it is recognized within that scope only. Hence, in a particular scope, a variable must have a unique name.
Constants
A constant represents a value that can’t be changed. In other words, a constant is a name that refers to a particular numeric, character, boolean, or string value.
Operators
Basically, an operator is a symbol that carries out a specific task. For example, operator + computes the sum of its operands. Furthermore, there are several categories of operators like arithmetic operators, relational operators, logical operators, assignment operators, and so on.
Strings
Likewise, a string is made up of characters that we enclose in either single quotation marks (”) or double quotation marks (” “).